티스토리 뷰
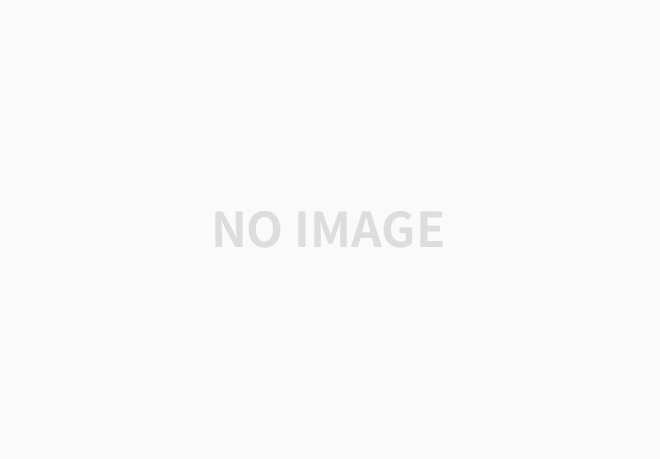
<!DOCTYPE HTML>
<html lang="ko">
<head>
<meta charset="utf-8">
<title>원형차트</title>
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta name="theme-color" content="#000000">
<script src="https://code.jquery.com/jquery-latest.js"></script>
<style>
/* 기본속성 */
* { margin: 0; padding: 0; box-sizing:border-box;}
/* root */
:root {
--circleLength: 220;
}
body { display: flex; align-items: center; justify-content: center; min-height: 100vh; background: #2f363e;}
#time { display: flex; gap: 30px;}
#time .circle { position: relative; width: 150px; height: 150px; display: flex; align-items: center; justify-content: center;}
#time .circle svg { position: relative; width: 150px; height: 150px; transform: rotate(90deg);}
#time .circle svg path { width: 100%; height: 100%; fill: transparent; stroke-width: 8; stroke: #282828; stroke-linecap: round; transform: translate(5px, 5px);}
/* stroke-dasharray: 점의 길이, stroke-dashoffset: 점 사이 간격의 길이 */
#time .circle svg path:nth-child(2) { stroke: var(--clr); stroke-dasharray: var(--circleLength); stroke-dashoffset: var(--circleLength); transition: all 0.5s;}
#time .oneValue { position: absolute; top: 30px; text-align: center; font-weight: 500; color: var(--clr); font-size: 1.5em;}
#time .oneValue span { position: absolute; transform: translateX(-50%) translateY(-10px); font-size: 0.35em; font-weight: 300; letter-spacing: 0.1em; text-transform: uppercase;}
#time .dots { transform: rotateZ(0deg); position: absolute; width: 100%; height: 100%; border-radius: 50%; display: flex; justify-content: center; align-items: flex-start; z-index: 1000; transition: all 0.5s;}
#time .dots::before { content: ''; position: absolute; top: 50%; left: -2px; width: 15px; height: 15px; margin-top: -7.5px; background: var(--clr); border-radius: 50%; box-shadow: 0 0 20px var(--clr), 0 0 60px var(--clr);}
#time .blind { position: absolute; bottom: 0; width: 100%; height: 50%; background-color: #2f363e;}
</style>
</head>
<body>
<div id="time">
<div class="circle" style="--clr: white;" data-graph='{"max":100, "value":70}'>
<div class="dots oneDot"></div>
<svg>
<path d="M 70,70 m 0, -70 a 70, 70, 0, 1, 0, -8.572527594031473e-15, 140"></path>
<path class="oneData" d="M 70,70 m 0, -70 a 70, 70, 0, 1, 0, -8.572527594031473e-15, 140"></path>
</svg>
<div class="oneValue"></div>
</div>
<div class="circle" style="--clr: yellow;" data-graph='{"max":100, "value":30}'>
<div class="dots oneDot"></div>
<svg>
<path d="M 70,70 m 0, -70 a 70, 70, 0, 1, 0, -8.572527594031473e-15, 140"></path>
<path class="oneData" d="M 70,70 m 0, -70 a 70, 70, 0, 1, 0, -8.572527594031473e-15, 140"></path>
</svg>
<div class="oneValue"></div>
</div>
</div>
<script>
let theme = document.querySelector(':root');
let themeStyles = getComputedStyle(theme);
let _circleValue = themeStyles.getPropertyValue('--circleLength');
var $item = $('.circle');
$.each($item, function(key, data){
var _dataValue = $item.eq(key).data('graph');
var _max = _dataValue.max;
var _value = _dataValue.value;
var _deg = 180 * _value/_max;
var _maxValue = Math.floor((_max * (_circleValue / _max)));
var _valueCost = _maxValue / (_max / _value);
$(data).find('.oneData').css('strokeDashoffset', Number(_circleValue) + _valueCost);
$(data).find('.oneDot').css('transform', `rotateZ(${_deg}deg)`);
var memberCountConTxt= _value;
$({ val : 0 }).animate({ val : memberCountConTxt }, {
duration: 500,
step: function() {
var num = numberWithCommas(Math.floor(this.val));
$(data).find('.oneValue').text(num);
},
complete: function() {
var num = numberWithCommas(Math.floor(this.val));
$(data).find('.oneValue').text(num);
}
});
// 콤마 필요시
function numberWithCommas(x) {
return x.toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",");
}
});
</script>
</body>
</html>
HTML 원형차트 jQuery
<!DOCTYPE HTML>
<html lang="ko">
<head>
<meta charset="utf-8">
<title>원형차트 HTML</title>
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, shrink-to-fit=no">
<meta name="theme-color" content="#000000">
<script src="https://code.jquery.com/jquery-latest.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/gsap/3.8.0/gsap.min.js"></script>
<style>
/* 기본속성 */
* { margin: 0; padding: 0; box-sizing:border-box;}
.p { margin-top: 30px; text-align: center;}
/* 차트 */
.circleFlex { display: flex; margin-top: 50px;}
.itemBody { position: relative; width: 100px; height: 100px; margin: 0 auto; background: #eee; border-radius: 50%;}
.item { position: absolute; top: 0; right: 0; bottom: 0; left: 0; border-radius: 50%;}
.item .circle { overflow: hidden; position: absolute; top: 0; right: 0; bottom: 0; left: 0; border-radius: 50%; font-size: 0; clip: rect(0, 100px, 100px, 50px); }
.item .bar { position: absolute; top: 0; right: 0; bottom: 0; left: 0; transition: transform .5s linear;}
.item .bar span { position: absolute; top: 0; left: -50%; width: 100%; height: 100%; background: #F36B6B; }
.item .bg { display: none; position: absolute; top: 0; right: 0; bottom: 0; left: 0; font-size: 0; border-radius: 50%; clip: rect(0, 100px, 1000px, 50px);}
.item .val { display: flex; flex-direction: column; align-items: center; justify-content: center; position: absolute; top: 10px; right: 10px; bottom: 10px; left: 10px; background: #fff; border-radius: 50%;}
.item.over .circle { clip: rect(0, 100px, 100px, 0);}
.item.over .bg { display: block; background: #F36B6B;}
</style>
</head>
<body>
<div id="wrap">
<div class="circleFlex">
<div class="itemBody">
<div class="item" data-graph='{"max":5, "value":1}'>
<div class="circle">
<span class="bar"><span></span></span>
<span class="bg"></span>
</div>
<div class="val">
<strong class="num"></strong>
<span>나쁨</span>
</div>
</div>
</div>
<div class="itemBody">
<div class="item" data-graph='{"max":10, "value":7}'>
<div class="circle">
<span class="bar"><span></span></span>
<span class="bg"></span>
</div>
<div class="val">
<strong class="num"></strong>
<span>좋음</span>
</div>
</div>
</div>
</div>
<p class="p">data-graph에 차트 값을 넣어준다. 예) {"max":10, "value":7}</p>
</div>
<script>
$(document).ready(function () {
circle = {
init: function () {
var $itemBody = $('.itemBody');
var $item = $itemBody.find('.item');
var $bar = $itemBody.find('.bar');
var _count = 0;
$.each($item, function(key, data){
var _dataValue = $item.eq(key).data('graph')
var _max = _dataValue.max;
var _value = _dataValue.value;
var _deg = 360 * _value / _max;
$itemBody.eq(key).find('.num').text(_value);
if(_deg > 180) {
$itemBody.eq(key).find('.bar').css({'transform':'rotate(180deg)'});
TweenLite.to($bar, 0.52, {onComplete:function() {
$itemBody.eq(key).find('.item').addClass('over');
$itemBody.eq(key).find('.bar').css({'transform':'rotate('+_deg+'deg)'});
}});
} else {
$itemBody.eq(key).find('.bar').css({'transform':'rotate('+_deg+'deg)'});
}
});
},
}
circle.init();
});
</script>
</body>
</html>
SVG 원형차트 jQuery
<!DOCTYPE HTML>
<html lang="ko">
<head>
<meta charset="utf-8">
<title>원형차트</title>
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta name="theme-color" content="#000000">
<script src="https://code.jquery.com/jquery-latest.js"></script>
<style>
/* 기본속성 */
* { margin: 0; padding: 0; box-sizing:border-box;}
/* root */
:root {
--circleLength: 440;
}
body { display: flex; align-items: center; justify-content: center; min-height: 100vh; background: #2f363e;}
#time { display: flex; gap: 30px;}
#time .circle { position: relative; width: 150px; height: 150px; display: flex; align-items: center; justify-content: center;}
#time .circle svg { position: relative; width: 150px; height: 150px; transform: rotate(270deg);}
#time .circle svg circle { width: 100%; height: 100%; fill: transparent; stroke-width: 8; stroke: #282828; transform: translate(5px, 5px);}
/* stroke-dasharray: 점의 길이, stroke-dashoffset: 점 사이 간격의 길이 */
#time .circle svg circle:nth-child(2) { stroke: var(--clr); stroke-dasharray: var(--circleLength); stroke-dashoffset: var(--circleLength); stroke-linecap: round; transition: all 0.5s;}
#time div { position: absolute; text-align: center; font-weight: 500; color: #555; font-size: 1.5em;}
#time div span { position: absolute; transform: translateX(-50%) translateY(-10px); font-size: 0.35em; font-weight: 300; letter-spacing: 0.1em; text-transform: uppercase;}
#time .dots { position: absolute; width: 100%; height: 100%; border-radius: 50%; display: flex; justify-content: center; align-items: flex-start; z-index: 1000; transition: all 0.5s;}
#time .dots::before { content: ''; position: absolute; top: -3px; width: 15px; height: 15px; background: var(--clr); border-radius: 50%; box-shadow: 0 0 20px var(--clr), 0 0 60px var(--clr);}
#time .oneValue { color: var(--clr);}
</style>
</head>
<body>
<div id="time">
<div class="circle" style="--clr: #fff;" data-graph='{"max":100, "value":75}'>
<div class="dots oneDot"></div>
<svg>
<circle cx="70" cy="70" r="70"></circle>
<circle cx="70" cy="70" r="70" class="oneData"></circle>
</svg>
<div class="oneValue">00</div>
</div>
<div class="circle" style="--clr: yellow;" data-graph='{"max":100, "value":30}'>
<div class="dots oneDot"></div>
<svg>
<circle cx="70" cy="70" r="70"></circle>
<circle cx="70" cy="70" r="70" class="oneData"></circle>
</svg>
<div class="oneValue">00</div>
</div>
</div>
<script>
let theme = document.querySelector(':root');
let themeStyles = getComputedStyle(theme);
let _circleValue = themeStyles.getPropertyValue('--circleLength');
var $item = $('.circle');
/*
oneData.style.strokeDashoffset = _circleValue - _valueCost;
theme.style.setProperty('--bg-color', 'green'); // 변수 값 변경
// 선택자 변수
element.style.getPropertyValue("--font-size");
element.style.setProperty("--font-size", 20);
*/
$.each($item, function(key, data){
var _dataValue = $item.eq(key).data('graph');
var _max = _dataValue.max;
var _value = _dataValue.value;
var _deg = 360 * _value/_max;
var _maxValue = Math.floor((_max * (_circleValue / _max)));
var _valueCost = _maxValue / (_max / _value);
$(data).find('.oneData').css('strokeDashoffset', _circleValue - _valueCost);
$(data).find('.oneDot').css('transform', `rotateZ(${_deg}deg)`);
var memberCountConTxt= _value;
$({ val : 0 }).animate({ val : memberCountConTxt }, {
duration: 500,
step: function() {
var num = numberWithCommas(Math.floor(this.val));
$(data).find('.oneValue').text(num);
},
complete: function() {
var num = numberWithCommas(Math.floor(this.val));
$(data).find('.oneValue').text(num);
}
});
function numberWithCommas(x) {
return x.toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",");
}
});
</script>
</body>
</html>
SVG 반원차트 jQuery
<!DOCTYPE HTML>
<html lang="ko">
<head>
<meta charset="utf-8">
<title>원형차트</title>
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta name="theme-color" content="#000000">
<script src="https://code.jquery.com/jquery-latest.js"></script>
<style>
/* 기본속성 */
* { margin: 0; padding: 0; box-sizing:border-box;}
/* root */
:root {
--circleLength: 220;
}
body { display: flex; align-items: center; justify-content: center; min-height: 100vh; background: #2f363e;}
#time { display: flex; gap: 30px;}
#time .circle { position: relative; width: 150px; height: 150px; display: flex; align-items: center; justify-content: center;}
#time .circle svg { position: relative; width: 150px; height: 150px; transform: rotate(90deg);}
#time .circle svg path { width: 100%; height: 100%; fill: transparent; stroke-width: 8; stroke: #282828; stroke-linecap: round; transform: translate(5px, 5px);}
/* stroke-dasharray: 점의 길이, stroke-dashoffset: 점 사이 간격의 길이 */
#time .circle svg path:nth-child(2) { stroke: var(--clr); stroke-dasharray: var(--circleLength); stroke-dashoffset: var(--circleLength); transition: all 0.5s;}
#time .oneValue { position: absolute; top: 30px; text-align: center; font-weight: 500; color: var(--clr); font-size: 1.5em;}
#time .oneValue span { position: absolute; transform: translateX(-50%) translateY(-10px); font-size: 0.35em; font-weight: 300; letter-spacing: 0.1em; text-transform: uppercase;}
#time .dots { transform: rotateZ(0deg); position: absolute; width: 100%; height: 100%; border-radius: 50%; display: flex; justify-content: center; align-items: flex-start; z-index: 1000; transition: all 0.5s;}
#time .dots::before { content: ''; position: absolute; top: 50%; left: -2px; width: 15px; height: 15px; margin-top: -7.5px; background: var(--clr); border-radius: 50%; box-shadow: 0 0 20px var(--clr), 0 0 60px var(--clr);}
#time .blind { position: absolute; bottom: 0; width: 100%; height: 50%; background-color: #2f363e;}
</style>
</head>
<body>
<div id="time">
<div class="circle" style="--clr: white;" data-graph='{"max":100, "value":70}'>
<div class="dots oneDot"></div>
<svg>
<path d="M 70,70 m 0, -70 a 70, 70, 0, 1, 0, -8.572527594031473e-15, 140"></path>
<path class="oneData" d="M 70,70 m 0, -70 a 70, 70, 0, 1, 0, -8.572527594031473e-15, 140"></path>
</svg>
<div class="oneValue"></div>
</div>
<div class="circle" style="--clr: yellow;" data-graph='{"max":100, "value":30}'>
<div class="dots oneDot"></div>
<svg>
<path d="M 70,70 m 0, -70 a 70, 70, 0, 1, 0, -8.572527594031473e-15, 140"></path>
<path class="oneData" d="M 70,70 m 0, -70 a 70, 70, 0, 1, 0, -8.572527594031473e-15, 140"></path>
</svg>
<div class="oneValue"></div>
</div>
</div>
<script>
let theme = document.querySelector(':root');
let themeStyles = getComputedStyle(theme);
let _circleValue = themeStyles.getPropertyValue('--circleLength');
var $item = $('.circle');
$.each($item, function(key, data){
var _dataValue = $item.eq(key).data('graph');
var _max = _dataValue.max;
var _value = _dataValue.value;
var _deg = 180 * _value/_max;
var _maxValue = Math.floor((_max * (_circleValue / _max)));
var _valueCost = _maxValue / (_max / _value);
$(data).find('.oneData').css('strokeDashoffset', Number(_circleValue) + _valueCost);
$(data).find('.oneDot').css('transform', `rotateZ(${_deg}deg)`);
var memberCountConTxt= _value;
$({ val : 0 }).animate({ val : memberCountConTxt }, {
duration: 500,
step: function() {
var num = numberWithCommas(Math.floor(this.val));
$(data).find('.oneValue').text(num);
},
complete: function() {
var num = numberWithCommas(Math.floor(this.val));
$(data).find('.oneValue').text(num);
}
});
// 콤마 필요시
function numberWithCommas(x) {
return x.toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",");
}
});
</script>
</body>
</html>
'HTML > 콤포넌트 모음' 카테고리의 다른 글
기타 등등 모음 (0) | 2023.07.03 |
---|---|
인풋 속성 정리 (0) | 2023.03.28 |
웹접근성을 지키는 Swiper (0) | 2021.11.25 |
웹접근성 지킨 라디오, 체크박스 (0) | 2021.11.24 |
버튼 (0) | 2021.11.22 |